How To Code a Brute-Force Script for Password Protected Zip Files - Python
Python has a build-in module zipfile which one can use for extracting zip files from their python code.
This how-to tutorial will show you how you can use this module to burte-force files with '.zip' extension.
Make sure you've a full access and permission for tasting this on files that does not belong to you, I'll be using a zip file that I created on my computer for demostration purpose.
import sys
We'll now create a function that will tell us if the current password in the wordlist is the correct password.
try:
zip_file.extractall(pwd=password)
return True
except Exception:
return False
In the above function, we accept two arguments, the first one(zip_file) is a ZipFile class object
which has many methods for working with zip files like close, open, getinfo, extract, extrall and more.
The second argument is the password to try.
In the extractall method, we passed in the password. If the password failed while extracting the file, it will
raise an exception which means the password isn't correct.
password_list = input("Enter password list file~$ ")
# If file name doesn't end with .zip we tell the user
# and quit the program
if not zip_file_name.endswith(".zip"):
print("Error Only '.zip' file supported")
exit(1)
# Initialize the ZipFile class
zip_file = zipfile.ZipFile(zip_file_name)
try:
with open(password_list, "r") as password:
for pass_w in password:
pass_w = pass_w.strip()
sys.stdout.write(f"{pass_w} ==> ")
if is_password(zip_file, pass_w):
sys.stdout.write("Found\n")
print(f"Password is {pass_w}")
exit(0)
sys.stdout.write("Not Found\n")
except Exception as err:
print(err)
except KeyboardInterrupt:
print("\nQuitting..")
exit(1)
In the above code, we get the zip file, and a wordlist path from the user, and we check
if the zip file doesn't ends with '.zip' Then we throw an error to the user.
zip_file = zipfile.ZipFile(zip_file_name) is use to Initialize the ZipFile class
and return the object to the zip_file,
Here is the defination of the ZipFile class
Full Source Code
import sys
def is_password(zip_file, password):
try:
zip_file.extractall(pwd=password)
return True
except Exception:
return False
zip_file_name = input(" Enter Zip file~$ ")
password_list = input(" Enter password list file~$ ")
# If file name doesn't end with .zip we tell the user
# and quit the program
if not zip_file_name.endswith(".zip"):
print("Error Only '.zip' file supported")
exit(1)
# Initialize the ZipFile class
zip_file = zipfile.ZipFile(zip_file_name)
try:
with open(password_list, "r") as password:
for pass_w in password:
pass_w = pass_w.strip()
sys.stdout.write(f"{pass_w} ==> ")
if is_password(zip_file, pass_w):
sys.stdout.write("Found\n")
print(f"Password is {pass_w}")
exit(0)
sys.stdout.write("Not Found\n")
except Exception as err:
print(err)
except KeyboardInterrupt:
print("\nQuitting..")
exit(1)
Now you need two things to teste the script, first thing
is a password protected zip file, then second is a password list.
After running the program, you should see something like this:
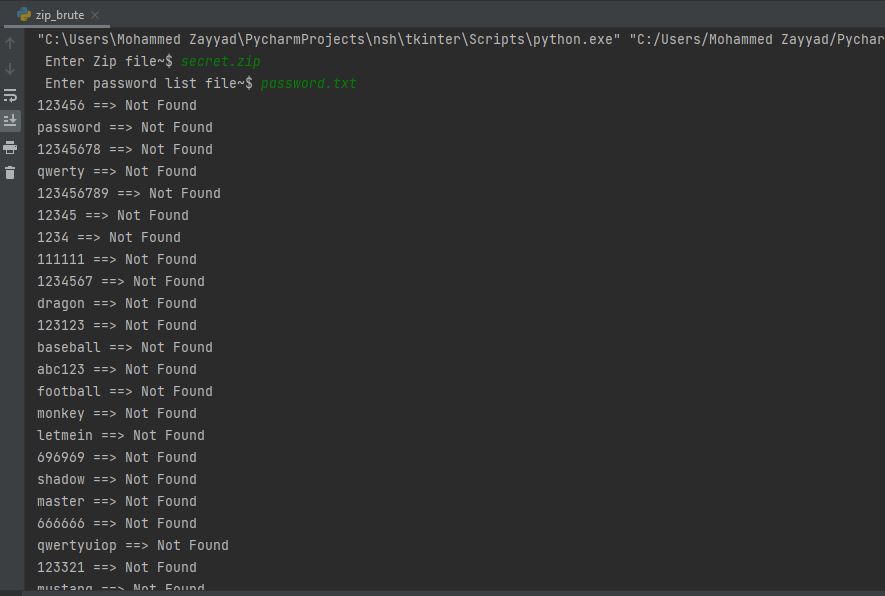